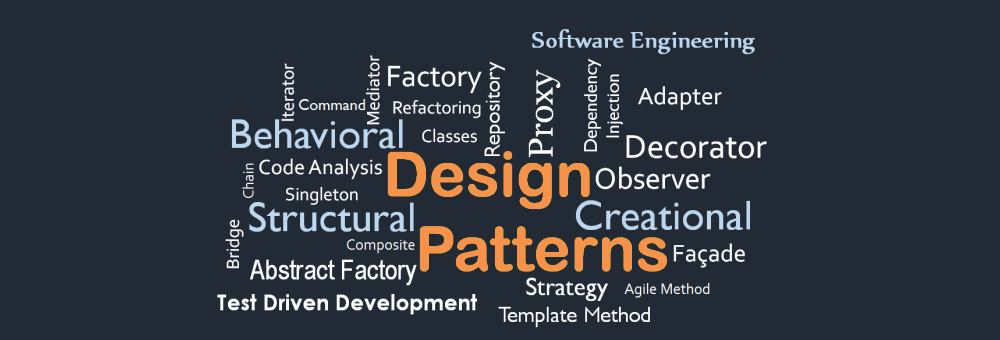
Introduction
Design patterns are reusable solutions to common software design problems. They are not specific to a particular programming language or technology but general concepts that can be applied to many different situations.
Design patterns can help you write code that is more modular, easier to understand, and easier to maintain. They can also help you avoid common pitfalls and mistakes leading to bugs and other issues.
There are many different design patterns, but they can be grouped into three main categories: creational, structural, and behavioral.
Creational Patterns
Creational patterns are used to create objects in a way that is flexible and easy to maintain. They include:
- Singleton: Ensures that only one instance of a class is created and provides a global point of access to that instance.
- Factory Method: Defines an interface for creating objects, but allows subclasses to decide which class to instantiate.
- Abstract Factory: Provides an interface for creating families of related objects without specifying their concrete classes.
- Builder: Separates the construction of a complex object from its representation, allowing the same construction process to create different representations.
Structural Patterns
Structural patterns are used to organize classes and objects in a way that is flexible and easy to understand. They include:
- Adapter: Allows two incompatible interfaces to work together by wrapping an object with a new interface.
- Bridge: Separates an object’s interface from its implementation, allowing them to vary independently.
- Composite: Allows you to treat a group of objects the same way you would treat a single object.
- Decorator: Allows you to add behavior to an object dynamically, without changing its interface.
- Facade: Provides a simplified interface to a complex system.
- Flyweight: Allows you to share objects to reduce memory usage.
Behavioral Patterns
Behavioral patterns are used to manage communication between objects in a way that is flexible and easy to understand. They include:
- Chain of Responsibility: Allows you to pass requests along a chain of objects until one of them handles the request.
- Command: Encapsulates a request as an object, allowing you to parameterize clients with different requests, queue or log requests, and support undoable operations.
- Interpreter: Defines the grammar of a language and provides an interpreter to interpret sentences in that language.
- Iterator: Provides a way to access the elements of an object sequentially without exposing its underlying representation.
- Mediator: Defines an object that encapsulates how a set of objects interact, allowing them to be decoupled from each other.
- Memento: Allows you to capture and restore an object’s internal state without violating encapsulation.
- Observer: Allows you to define a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically.
- State: Allows an object to alter its behavior when its internal state changes.
- Strategy: Defines a family of algorithms, encapsulates each one, and makes them interchangeable.
- Template Method: Defines the skeleton of an algorithm in a method, allowing subclasses to provide specific behavior for certain steps.
- Visitor: Allows you to add new operations to an object structure without changing the objects.
Conclusion
Design patterns are a powerful tool for software developers. By using them, you can write code that is more modular, easier to understand, and easier to maintain. There are many different design patterns to choose from, and each one has its own strengths and weaknesses. By understanding the different types of design patterns and when to use them, you can become a more effective and efficient programmer.